Docker MongoDB Container
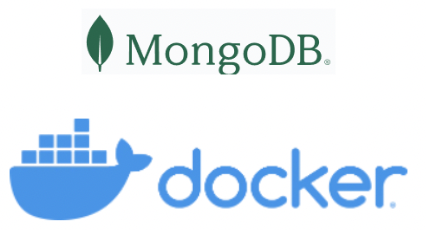
Are you looking to set up a MongoDB container using Docker on your MacOS machine? Look no further! In this article, we'll walk you through the process of creating and configuring a Docker MongoDB container, including setting environment variables, starting the container, and testing the database.
Step 1: Create an Environment File
The first step is to create an environment file that will store our database password.
.env
DB_PASSWORD=Password1234
This file will be used to set the MONGO_INITDB_ROOT_PASSWORD
environment variable in our Docker container.
Step 2: Create a Docker Compose File
Next, we'll create a Docker compose file that defines our MongoDB container.
mongo-docker-compose.yml
version: '3.9'
services:
mongo-db:
# Apple M1 Chip
# platform: linux/amd64
image: mongo:4.4.18
container_name: mongo-db
restart: always
env_file:
- .env
environment:
MONGO_INITDB_ROOT_USERNAME: root
MONGO_INITDB_ROOT_PASSWORD: $DB_PASSWORD
ports:
- 27019:27017
volumes:
- mongo_datadir:/data/db
networks:
- backend-network
networks:
backend-network:
driver: bridge
volumes:
mongo_datadir:
This file defines a single service, mongo-db, which uses the official MongoDB 4.4.18 image and maps port 27019 on your local machine to port 27017 in the container.
Step 3: Start the Container
Now that we have our environment file and Docker compose file set up, it's time to start the container! Run the following command:
Now we can start the container.
docker-compose -f mongo-docker-compose.yml up -d
This will start the container in detached mode, meaning it will run in the background.
Step4: Test MongoDB Database Container
Once the container is running, we can test our database by creating a collection & some documents. Run the following commands:
Let us create a sample javascript file to create -
loadMovies.js
db = connect( 'mongodb://root:Password1234@127.0.0.1:27019/films?authSource=admin' );
db.movies.insertMany( [
{
title: 'Titanic',
year: 1997,
genres: [ 'Drama', 'Romance' ]
},
{
title: 'Spirited Away',
year: 2001,
genres: [ 'Animation', 'Adventure', 'Family' ]
},
{
title: 'Casablanca',
genres: [ 'Drama', 'Romance', 'War' ]
}
] )
Now we will create a database and some tables.
Note: you should have MongoDB Shell Client installed locally.
db_password=Password1234
mongosh --host 127.0.0.1 --port 27019 --username root --password ${db_password} --authenticationDatabase admin --file loadMovies.js
Test the database objects-
readMovies.js
db = connect( 'mongodb://root:Password1234@127.0.0.1:27019/films?authSource=admin' );
printjson( db.movies.find( {} ) );
mongosh --host 127.0.0.1 --port 27019 --username root --password ${db_password} --authenticationDatabase admin --file readMovies.js
Step 5: Stop the Container
Finally, we can stop the container by running the following command:
docker-compose -f mongo-docker-compose.yml down
This will stop the container and remove it from memory.
That's it! We've successfully created and configured a Docker MongoDB container.